Simple Reservation Calendar with first-class Vue support
Zaptime offers an embeddable, feature-rich, TypeScript-ready, SSR-friendly calendar solution designed to integrate with Vue and Nuxt applications. It allows developers to easily customize appearance, build custom UI layer and much more.
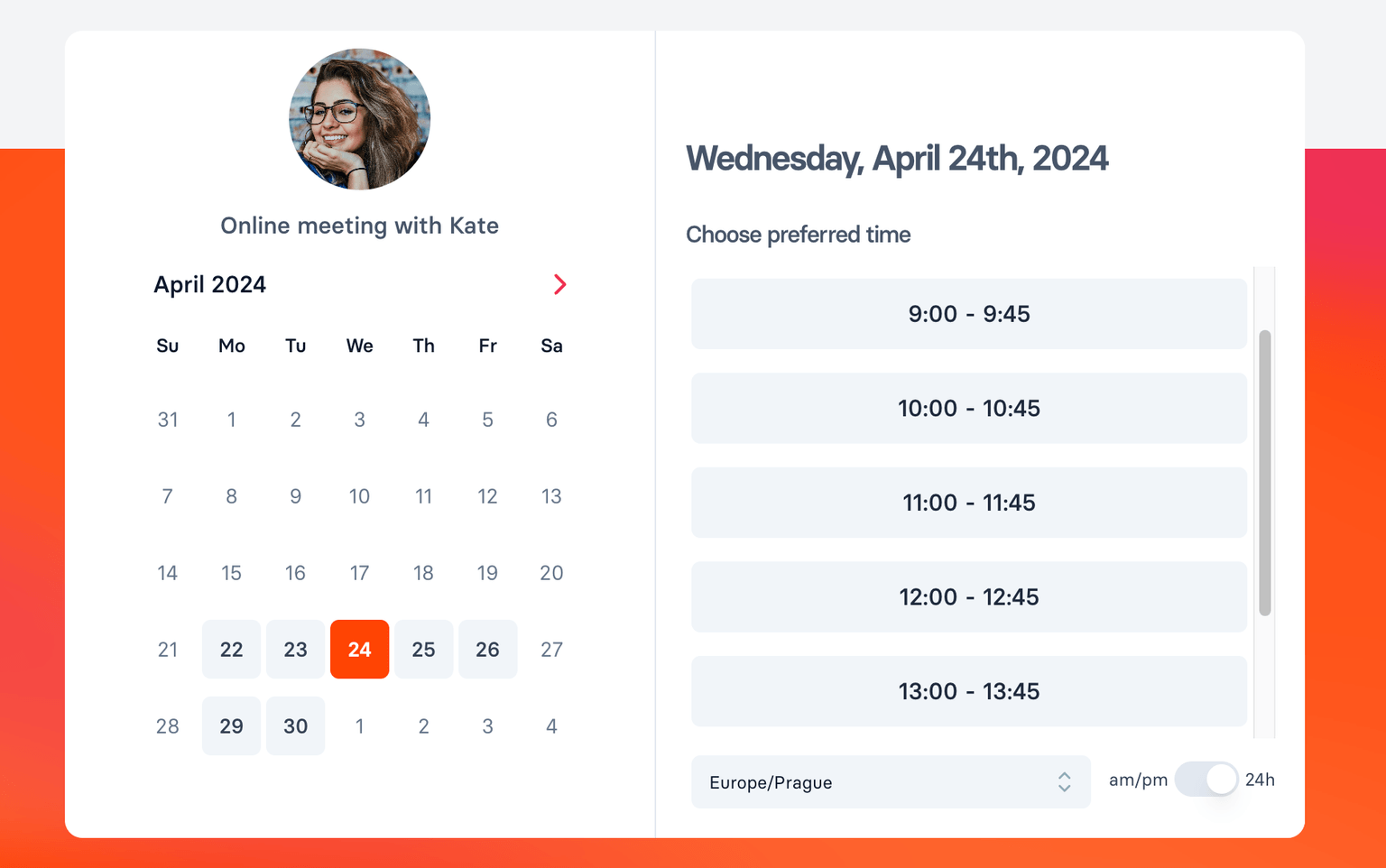
Simple Integration
This is how easy it is to get a default calendar up and running.
<template>
<ZaptimeCalendar :config="config" />
</template>
<script setup lang="ts">
import { ZaptimeCalendar } from "@zaptime/vue3";
import type { ZaptimeConfig } from "@zaptime/vue3";
const config: ZaptimeConfig = {
token: "<API_TOKEN>",
};
</script>
Layer by Layer customizations
By utilizing composables, developers can easily tap into the core functionalities of Zaptime, enhancing the calendar's capabilities without the hassle of extensive configurations. Control the state of the calendar, watch for state changes inside the Calendar and more.
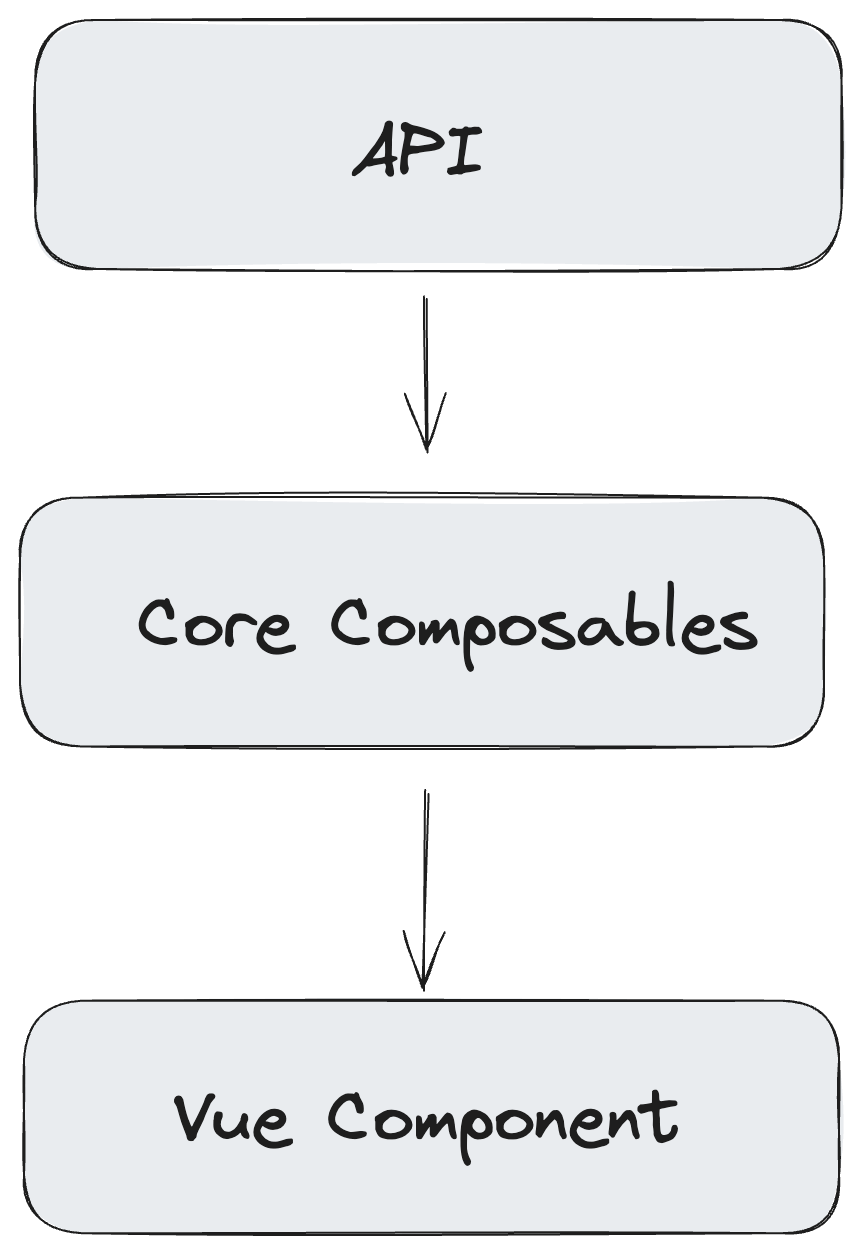
API documentation
Composables documentation
Make the Calendar yours
Zaptime’s architecture supports extensive customization, from the visual aspects to the functional interactions. Use built-in Vue composables to get all data needed to build a custom calendar. And build the UI yourself.
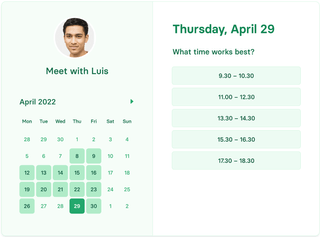
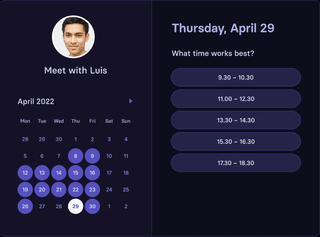
Play with Zaptime StackBlitz integration example.
Direct access to Calendar Data
Blend Zaptime directly into your existing forms allowing you to manage and manipulate calendar data directly within your application workflows. This ensures that all scheduling features are tightly aligned with your user interface, enhancing your event handling processes.
<template>
<ZaptimeCalendar :config="config" />
</template>
<script setup lang="ts">
import { ZaptimeCalendar } from "@zaptime/vue3";
import type { ZaptimeConfig } from "@zaptime/vue3";
import { useCalendar, useSelectedTimeSlot } from "@zaptime/core";
// Access internal calendar state
const { state } = useCalendar();
// Retrieve currently selected time slot
const { selectedTimeSlot } = useSelectedTimeSlot();
const config: ZaptimeConfig = {
token: "<API_TOKEN>",
};
</script>
Never Double Book
Zaptime includes a feature that allows you to reserve time slots pending confirmation, providing flexibility and ensuring that time slots are never double-booked. This is particularly valuable when integrating with payment systems, as it secures the slot while awaiting transaction completion, reducing the risk of scheduling conflicts.
<script setup lang="ts">
import { reserve, confirm } from "@zaptime/vue3";
async function submitForm() {
await reserve();
/*
Do whatever you need, reserved time slot
is pending confirmation for 15 minutes.
*/
await confirm();
}
</script>
Easy Installation and Documentation
Getting started with Zaptime is as simple as installing the NPM package. The Vue component can be integrated with minimal setup and additional features can be incrementally added when needed.
Play with Zaptime StackBlitz integration example.
For complete documentation check our developer's docs.